Create or update Riffusion API account configuration
February 17, 2025 (February 21, 2025)
Riffussion API has been decommissioned. Currently we do not have plans to re-release it and recommend switching to Mureka API for music generation.
Table of contents
After you have completed the steps defined in Setup Riffusion and retrieved your cookie, you can use this endpoint to set up your Riffusion API account.
It is very important that you execute this endpoint with a fresh cookie only ONCE. If you attempt to post the same cookie twice, the API will not be able to refresh it, even though the request will be accepted.
Once you’ve added your account, we recommend leaving it as is and letting the API manage the session. To do this, you can either close the browser tab or, preferably, reset your current browser session by clearing the cookies under the Dev Tools » Application tab and refreshing your browser to acquire a new session.
You can also execute the script below to clear all the cookies.
Script to clear all the cookies
Use the handy script below to clear all session cookies. This will make your browser forget the current session—think of it as a soft logout.
document.cookie.split(';').forEach(cookie => {
const name = cookie.split('=')[0].trim();
document.cookie = `${name}=;expires=Thu, 01 Jan 1970 00:00:00 UTC;path=/`;
});
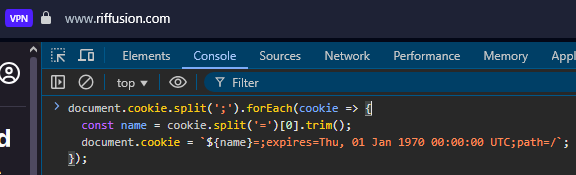
https://api.useapi.net/v1/riffusion/accounts
Request Headers
Authorization: Bearer {API token}
Content-Type: application/json
API token
is required, see Setup useapi.net for details.
Request Body
{
"cookie": "Riffusion cookie",
"maxJobs": 1-10,
}
-
cookie
is required. Please see Setup Riffusion for details. -
maxJobs
is required. Currently, it should be between 1 and 10.
We recommend setting this value to 6.
Responses
-
{ "user_id": "b511d6f4-1e33-45c3-885d-0fa094dd5d70", "maxJobs": 6, "jwt": { "expires_at": 1781234765, "expires_in": 604800, "refresh_token": "…secured…", "access_token": "…secured…", "expires_at_UTC": "2025-04-18T12:50:45.000Z", "token_type": "bearer", "user": { "id": "b511d6f4-1e33-45c3-885d-0fa094dd5d70" } } }
-
{ "error": "<Error message>", "code": 400 }
Model
{ // TypeScript, all fields are optional
user_id: string
maxJobs: number
jwt: {
access_token: string
token_type: string
expires_in: number
expires_at: number
refresh_token: string
expires_at_UTC: string
user: {
id: string
}
}
}
Examples
-
curl -H "Accept: application/json" \ -H "Content-Type: application/json" \ -H "Authorization: Bearer …" \ -X POST https://api.useapi.net/v1/riffusion/accounts \ -d '{"cookie": "…", "maxJobs": …}'
-
const apiUrl = `https://api.useapi.net/v1/riffusion/accounts`; const api_token = "API token"; const cookie = "Riffusion cookie"; const maxJobs = 6; const data = { method: 'POST', headers: { 'Authorization': `Bearer ${api_token}`, 'Content-Type': 'application/json' } }; data.body = JSON.stringify({ cookie, maxJobs }); const response = await fetch(apiUrl, data); const result = await response.json(); console.log("response", {response, result});
-
import requests apiUrl = f"https://api.useapi.net/v1/riffusion/accounts" api_token = "API token" cookie = "Riffusion cookie" maxJobs = 6 headers = { "Content-Type": "application/json", "Authorization" : f"Bearer {api_token}" } body = { "cookie": f"{cookie}", "maxJobs": maxJobs } response = requests.post(apiUrl, headers=headers, json=body) print(response, response.json())